Introduction to Mastering Pseudocode
Pseudocode serves as a crucial tool that simplifies the representation of algorithms without adhering to syntax rules of specific programming languages. This article delves into the nuances of developing succinct and effective pseudocode that bridges the conceptual journey from problem-solving to coding.
The Essence of Pseudocode
Pseudocode’s primary role is to outline an algorithm’s design, facilitating a smooth translation to actual code by offering a medium that focuses on logic rather than language-specific syntax.
Key Structural Elements
Effective pseudocode incorporates structured linguistic elements that ease the conversion to programmable code, which includes variable declarations, control structures, subroutines, and commentary for clarification.
Excelling in Pseudocode Creation
To excel in pseudocode, it is vital to ensure clarity, consistency, language neutrality, and attentiveness to details for seamless code translation.
Developing Pseudocode Step-by-Step
Comprehending the Problem
Begin with a complete understanding of the problem, breaking it down into smaller segments for better manageability.
Defining the Desired Outcome
The algorithm’s expected output should be clearly defined as it will guide the direction of the pseudocode.
Selecting Key Variables and Structures
Determine the essential variables and choose data structures that effectively handle the algorithm’s data.
Algorithm Construction
Initiate with broad instructions that gradually refine into detailed steps, ensuring logical arrangement throughout.
Iterative Enhancement
Persistently refine your pseudocode to eliminate ambiguities or superfluous elements, striving for clarity in each statement.
Evaluating and Testing
Ensure that your pseudocode addresses every aspect of the problem and conduct dry runs to validate logic and functionality.
Advancing Your Pseudocode Skills
As you gain proficiency, incorporate advanced concepts like modularity, data abstraction, and established algorithmic paradigms to enhance your pseudocode.
Illustrative Pseudocode Examples
Linear Search Algorithm Example
// Pseudocode for a Linear Search to locate a value within an array.
DEFINE FUNCTION LinearSearch(Array, ValueToFind)
FOR Index IN 0 TO Array.Length - 1
IF Array[Index] IS ValueToFind THEN
RETURN Index
ENDIF
NEXT
RETURN -1 // Value not found
END FUNCTION
Bubble Sort Algorithm Example
// Pseudocode for Bubble Sort to order an array in ascending sequence.
DEFINE FUNCTION BubbleSort(Array)
REPEAT
Swapped = false
FOR Index IN 0 TO Array.Length - 2
IF Array[Index] > Array[Index+1] THEN
SWAP Array[Index], Array[Index+1]
Swapped = true
ENDIF
NEXT
UNTIL NOT Swapped
END FUNCTION
Customizing Pseudocode for Various Scenarios
Adapt your pseudocode to fit particular use cases, such as data analysis, AI applications, or database processes, tailoring detail and structure to match task complexity.
Conclusion
Mastering pseudocode is a fundamental step in software development, paving the way for efficient and precise programming by adhering to principles of simplicity and transparency.
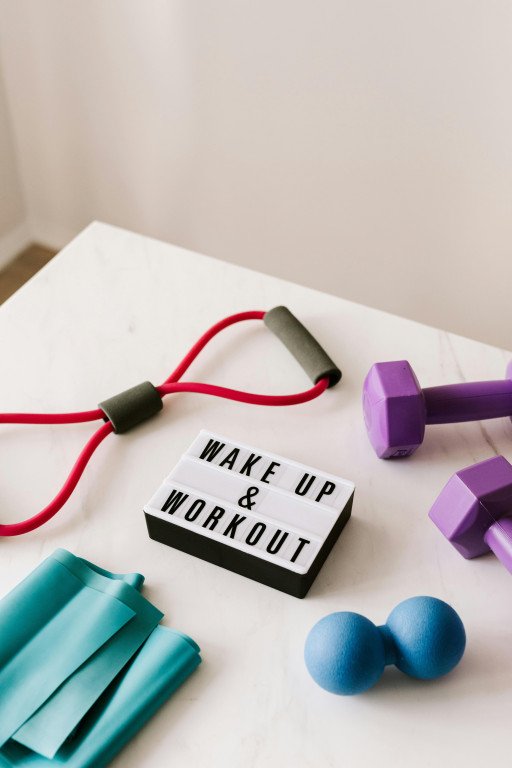
Wikipedia offers a comprehensive overview of pseudocode for those seeking a more in-depth exploration.
paper coding for programmers essential mastery techniques
Related Posts
- 10 Effective Steps for Data Structures and Algorithms Mastery using LeetCode: An In-Depth Guide
- Insertion Sort in Python: 5 Essential Steps for Efficient Coding
- Python Commenting Techniques: 7 Tips for Better Code Documentation
- Paper Coding for Programmers: 7 Essential Mastery Techniques
- 5 Essential Tips to Master Merge Sort in Java