Introduction to the Merge Sort Algorithm
In computational science, sorting algorithms are crucial for efficient data organization, and Merge Sort particularly excels with its O(n log n) time complexity. This method operates on a divide-and-conquer strategy to ensure stable, consistent sorting of data structures.
The Mechanics Behind Merge Sort
Merge Sort Java Guide starts by dividing an array until each part consists of a single element. These parts are then merged in a specific order, gradually building up to the fully sorted array.
Guide to Implementing Merge Sort in Java
Understanding how to harness Merge Sort Java Guide in your applications is critical. Below is a simplified guide:
Step 1: Divide the Array
The merge sort process begins by recursively dividing the array into smaller sections.
private void mergeSort(int[] array, int left, int right) { // Code omitted for brevity, see full function implementation below }
Step 2: Converge the Subsections
After division, merging combines these ordered subsections into a unified array.
private void merge(int[] array, int left, int middle, int right) { // Code snippet demonstrates the merging process // Full code details are available in the step-by-step guide }
Step 3: Synthesize the Techniques
By integrating both division and merger, we establish a comprehensive Merge Sort routine.
public class MergeSort { // Relevant methods included here elucidate the complete Merge Sort logic // Main and helper methods illustrate the Merge Sort usage }
Optimizing Merge Sort for Practical Use
To further enhance Merge Sort’s efficacy in real-world scenarios, one could minimize resource consumption or employ hybrid strategies.
Comparative Analysis: Merge Sort Against Other Algorithms
When juxtaposed with alternatives like Quick Sort, Merge Sort showcases notable advantages in stability and utility, such as in linked list organization and external sorting tasks.
Concluding Thoughts on Merge Sort in Java
Proficiency in Merge Sort Java Guide can significantly bolster your data structuring and sorting prowess, readying you for advanced algorithmic challenges.
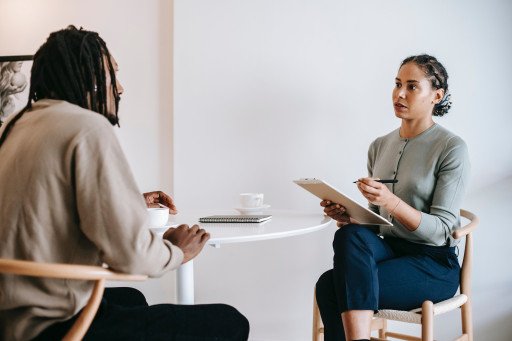
For further enhancement on data structures and algorithm proficiency, do consider exploring effective steps data structures algorithms mastery leetcode guide.
Find more insights and best practices on sorting algorithms from trusted resources on the web. For example, the Wikipedia article on Merge Sort is a valuable reference for understanding historical context and technical specifics.