Unlocking the Basics of Insertion Sort in C
An integral algorithm for programmers, Insertion Sort in C, offers a straightforward yet powerful method to organize data one step at a time. Ideal for small datasets or when more sophisticated sorting methods like quicksort are not required, this algorithm has its unique set of advantages.
How Insertion Sort Operates
In practice, this algorithm painstakingly moves elements from an unsorted collection into the correct spots within a new, orderly list. Initially empty, the sorted section grows progressively as elements find their rightful place, ensuring an organized sequence emerges.
Methodical Process of Insertion Sort
Familiarize yourself with these sequential steps:
- Begins with the array’s second element, as the first is presumed sorted.
- Each element is scrutinized and compared against those in the sorted array to determine its destination.
- Meanwhile, elements bigger than the new addition shuffle to the right, opening up necessary space.
- With precision, the element is placed into its newfound home.
- This process repeats for every single element within the list.
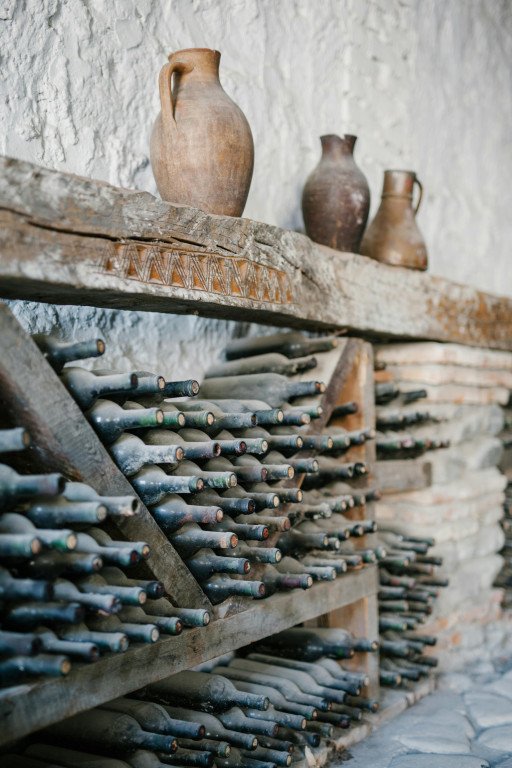
Optimization Techniques for Insertion Sort in C
To streamline the Insertion Sort in C process, adopt best practices such as minimizing both assignments and comparisons, judiciously utilizing loop constructs, and introducing sentinel values to curtail operations within the inner loop.
Insertion Sort Explained With Code
The following C implementation elucidates insertion sort’s simplicity:
#include <iostream>
using namespace std;
void insertionSort(int arr[], int length) {
int i, key, j;
for (i = 1; i < length; i++) {
key = arr[i];
j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
int main() {
int arr[] = {12, 11, 13, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
insertionSort(arr, n);
cout << "Sorted array: \n";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
return 0;
}
Evaluating Insertion Sort’s Complexity
With a nested loop structure, Insertion Sort in C has an O(n^2) time complexity, although real-world performance may be more favorable with pre-sorted inputs. It also maintains excellent space efficiency, requiring only O(1) additional storage.
The Advantages of Insertion Sort:
- Splendid for modest-sized data.
- Transcends bubble sort and selection sort in efficiency.
- Adapts quickly, performing less work as it nears sortedness.
- Stability is a hallmark, preserving element order with identical keys.
- Minimalistic memory footprint makes it an in-place sort.
- Capable of sorting live, incrementally received data.
Discover key aspects of data structures and efficient computing.
Practical Realms for Insertion Sort
- Online sorting of incremental arrays.
- Sorting live data streams with ongoing interactions.
- Environments where conserving memory and simplicity are paramount.
In Summary: Embracing Insertion Sort for Effective C Programming
Leveraging the distinct benefits offered by the Insertion Sort in C can prove invaluable, particularly when confronting small or almost ordered data arrays. Mastery of this algorithm is not just practical but marks a significant proficiency in any programmer’s skillset.
Related Posts
- 5 Essential Insights from a Data Structures and Algorithms Guide
- Comprehensive Guide to Data Structures and Algorithms
- 5 Strategies for Greedy Method Algorithm Optimization in Various Applications
- 7 Key Aspects of Data Structures and Efficient Computing
- 5 Key Insights into the Nelder-Mead Optimization Technique